Developer Libraries
Third-party Integrations
Best DNS Lookup SDK: Seamlessly Integrate WhoisFreaks APIs with Go
The SDK allows you to perform live, historical, and reverse DNS lookups. You can retrieve comprehensive domain name system records, which are essential for network analysis, troubleshooting, and ensuring proper domain configurations. This feature is ideal for developers managing DNS data and conducting network diagnostics.
DNS Lookups
This package is for performing any type of DNS lookup, such as live, historical, or reverse lookups.
To utilize the WhoisFreaks Go SDK for DNS Lookups of domain names or IP addresses, follow these steps:
-
DNS Authentication Setup
To authenticate your API requests, set your API key using the SetAPIKey method provided by the SDK. This method sets the global API key to the specified value.
bash Copyfunc SetAPIKey(key string)
Parameter:
- key: A string representing the API key to be set.
Example Usage:
go Copypackage main import ( "github.com/WhoisFreaks/whoisfreaks/whois" ) func main() { whois.SetAPIKey("your_api_key") // Your code here }
Replace "your_api_key" with your actual API key.
-
DNS Live Lookup
GetLiveResponse performs a live DNS lookup using the WhoisFreaks API. It retrieves real-time DNS information for a specific domain and DNS type.
go Copyfunc GetLiveResponse(dnsType, domain string) (*modal.DNSInfo, *modal.Error)
Parameters:
- dnsType: The type of DNS record to look up (e.g., "A", "MX", "CNAME", "AAAA", "NS", "TXT", "SOA", "SPF").
- domain: The domain name for which live DNS information is requested.
Returns:
- *modal.DNSInfo: A pointer to a DNSInfo struct containing live DNS information.
- *modal.Error: A pointer to an Error struct if there is an API error, or nil if the request is successful.
Example Usage:
go Copypackage main import ( "fmt" "log" "github.com/WhoisFreaks/whoisfreaks/dns" ) func main() { dns.SetAPIKey("your_api_key") domain := "example.com" dnsType := "A" // Replace with desired DNS record type (e.g., "A", "MX", "CNAME", "AAAA", "NS", "TXT", "SOA", "SPF") result, err := dns.GetLiveResponse(dnsType, domain) if err != nil { log.Fatal(err) } fmt.Println(result) }
Replace domain with the domain you wish to query and dnsType with the desired DNS record type (e.g., "A", "MX", "CNAME").
-
DNS Historical Lookup
GetHistoricalResponse performs a historical DNS lookup using the WhoisFreaks API. It retrieves historical DNS information for a specific domain and DNS type.
go Copyfunc GetHistoricalResponse(dnsType, domain, page string) (*modal.HistoricalDnsInfo, *modal.Error)
Parameters:
- dnsType: The type of DNS record to look up (e.g., "A", "MX", "CNAME", "AAAA", "NS", "TXT", "SOA", "SPF").
- dnsType: The domain name for which historical DNS information is requested.
- page: The optional page number for paginated results. Leave empty for the first page.
Returns:
- *modal.HistoricalDnsInfo: A pointer to a HistoricalDnsInfo struct containing historical DNS information.
- *modal.Error: A pointer to an Error struct if there is an API error, or nil if the request is successful.
Example Usage:
go Copypackage main import ( "fmt" "log" "github.com/WhoisFreaks/whoisfreaks/dns" ) func main() { dns.SetAPIKey("your_api_key") domain := "example.com" dnsType := "A" // Replace with desired DNS record type page := "1" // Optional: specify the page number for paginated results result, err := dns.GetHistoricalResponse(dnsType, domain, page) if err != nil { log.Fatal(err) } fmt.Println(result) }
Replace "example.com" with the domain you wish to query, adjust dnsType as needed, and specify the page number if necessary.
-
DNS Reverse Lookup
GetReverseResponse performs a reverse DNS lookup using the WhoisFreaks API.
go Copyfunc GetReverseResponse(dnsType, value, page string) (*modal.ReverseDnsInfo, *modal.Error)
Parameters:
- dnsType: The type of DNS record to look up (e.g., "a"). Multiple records are not supported.
- value: The IP address or value for which reverse DNS information is requested.
- page: The optional page number for paginated results. Leave empty for the first page.
Returns:
- *modal.ReverseDnsInfo: A pointer to a ReverseDnsInfo struct containing reverse DNS information.
- *modal.Error: A pointer to an Error struct if there is an API error, or nil if the request is successful.
Example Usage:
go Copypackage main import ( "fmt" "log" "github.com/WhoisFreaks/whoisfreaks/dns" ) func main() { dns.SetAPIKey("your_api_key") ipAddress := "8.8.8.8" // Replace with the IP address you wish to query dnsType := "A" // Replace with desired DNS record type page := "1" // Optional: specify the page number for paginated results result, err := dns.GetReverseResponse(dnsType, ipAddress, page) if err != nil { log.Fatal(err) } fmt.Println(result) }
Replace "8.8.8.8" with the IP address you wish to query, adjust dnsType as needed, and specify the page number if necessary.
Click here if you want to know how to perform WHOIS lookups using the Go SDK.
Click here to find out how to check domain availability using the Go SDK.
Click here if you want to learn how to perform SSL certificate lookups using the Go SDK.
Explore the official WhoisFreaks Go SDK documentation for DNS record lookups and management.
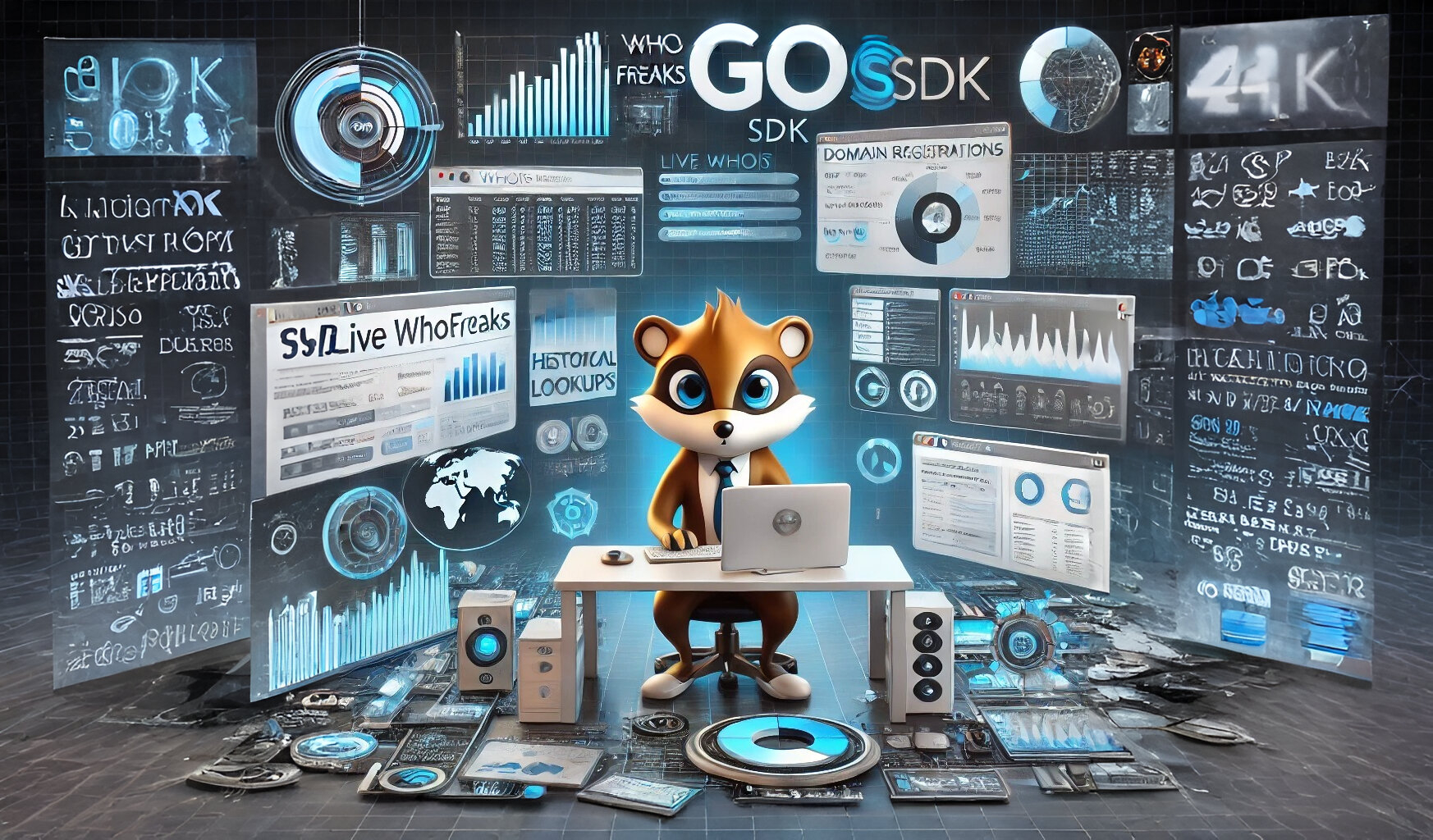
GoLang DNS Lookups SDK
Use this Go Lang-based SDK tool to effortlessly integrate with the WhoisFreaks DNS API for real-time DNS record queries.
pkg.go.dev