Developer Libraries
Third-party Integrations
Domain Availability Lookup SDK: Integrate WhoisFreaks APIs using Go
The WhoisFreaks Go SDK enables you to check the availability status of domain names, helping developers determine whether a domain is available for registration. This feature supports domain acquisition, brand protection, and proactive management of digital assets by identifying potential domain opportunities.
Domain Availability Lookup
This package is for performing any type of Domain Availability Lookup.
To utilize the WhoisFreaks Go SDK for checking the availability of domain names, follow these steps:
-
Domain Availability Authentication Setup
To authenticate your API requests, set your API key using the SetAPIKey method provided by the SDK. This method sets the global API key to the specified value.
bash Copyfunc SetAPIKey(key string)
Parameter:
- key: A string representing the API key to be set.
Example Usage:
go Copypackage main import ( "github.com/WhoisFreaks/whoisfreaks/whois" ) func main() { whois.SetAPIKey("your_api_key") // Your code here }
Replace "your_api_key" with your actual API key.
-
Domain Availability Check
Check performs a domain availability check using the WhoisFreaks API. It checks whether a specific domain name is available for registration.
go Copyfunc Check(domain string) (*modal.DomainAvailability, *modal.Error)
Parameter:
- domain: The domain name to be checked for availability (e.g., "example.com").
Returns:
- *modal.DomainAvailability: A pointer to a DomainAvailability struct containing domain availability information.
- *modal.Error: A pointer to an Error struct if there is an API error, or nil if the request is successful.
Example Usage:
go Copypackage main import ( "fmt" "log" "github.com/WhoisFreaks/whoisfreaks/domainavailability" ) func main() { domainavailability.SetAPIKey("your_api_key") domain := "example.com" result, err := domainavailability.Check(domain) if err != nil { log.Fatal(err) } fmt.Println(result) }
Replace "example.com" with the domain you wish to check.
-
Domain Availability Check and Suggestion
CheckAndSuggest performs a domain availability check and suggests similar domain names using the WhoisFreaks API.
go Copyfunc CheckAndSuggest(domain string, sug bool, count string) (*modal.BulkDomainAvailability, *modal.Error)
Parameters:
- domain: The domain name to be checked for availability (e.g., "example.com").
- sug: A boolean flag indicating whether to suggest similar domain names.
- count: The number of suggested domain names to retrieve (valid only if sug is true).
Returns:
- *modal.DomainAvailability: A pointer to a DomainAvailability struct containing domain availability information.
- *modal.Error: A pointer to an Error struct if there is an API error, or nil if the request is successful.
Example Usage:
go Copypackage main import ( "fmt" "log" "github.com/WhoisFreaks/whoisfreaks/domainavailability" ) func main() { domainavailability.SetAPIKey("your_api_key") domain := "example.com" suggestions := true count := "5" // Number of suggestions result, err := domainavailability.CheckAndSuggest(domain, suggestions, count) if err != nil { log.Fatal(err) } fmt.Println(result) }
Replace "example.com" with the domain you wish to check, set suggestions to true to enable suggestions, and specify the number of suggestions with count.
-
Bulk Domain Availability Lookup
Bulk performs a bulk domain availability check using the WhoisFreaks API. It checks the availability of multiple domain names in bulk.
go Copyfunc Bulk(domains []string) (*modal.BulkDomainAvailability, *modal.Error)
Parameter:
- domains: A slice of strings containing the domain names to be checked for availability.
Returns:
- *modal.BulkDomainAvailability: A pointer to a BulkDomainAvailability struct containing bulk domain availability information.
- *modal.Error: A pointer to an Error struct if there is an API error, or nil if the request is successful.
Example Usage:
go Copypackage main import ( "fmt" "log" "github.com/WhoisFreaks/whoisfreaks/domainavailability" ) func main() { domainavailability.SetAPIKey("your_api_key") domains := []string{"example1.com", "example2.com", "example3.com"} result, err := domainavailability.Bulk(domains) if err != nil { log.Fatal(err) } fmt.Println(result) }
Replace the domain names in the domains slice with the domains you wish to check.
Click here if you want to know how to perform WHOIS lookups using the Go SDK.
Click here for instructions on performing DNS lookups with the Go SDK.
Click here if you want to learn how to perform SSL certificate lookups using the Go SDK.
Check out the official WhoisFreaks Go SDK documentation for domain availability lookups and management.
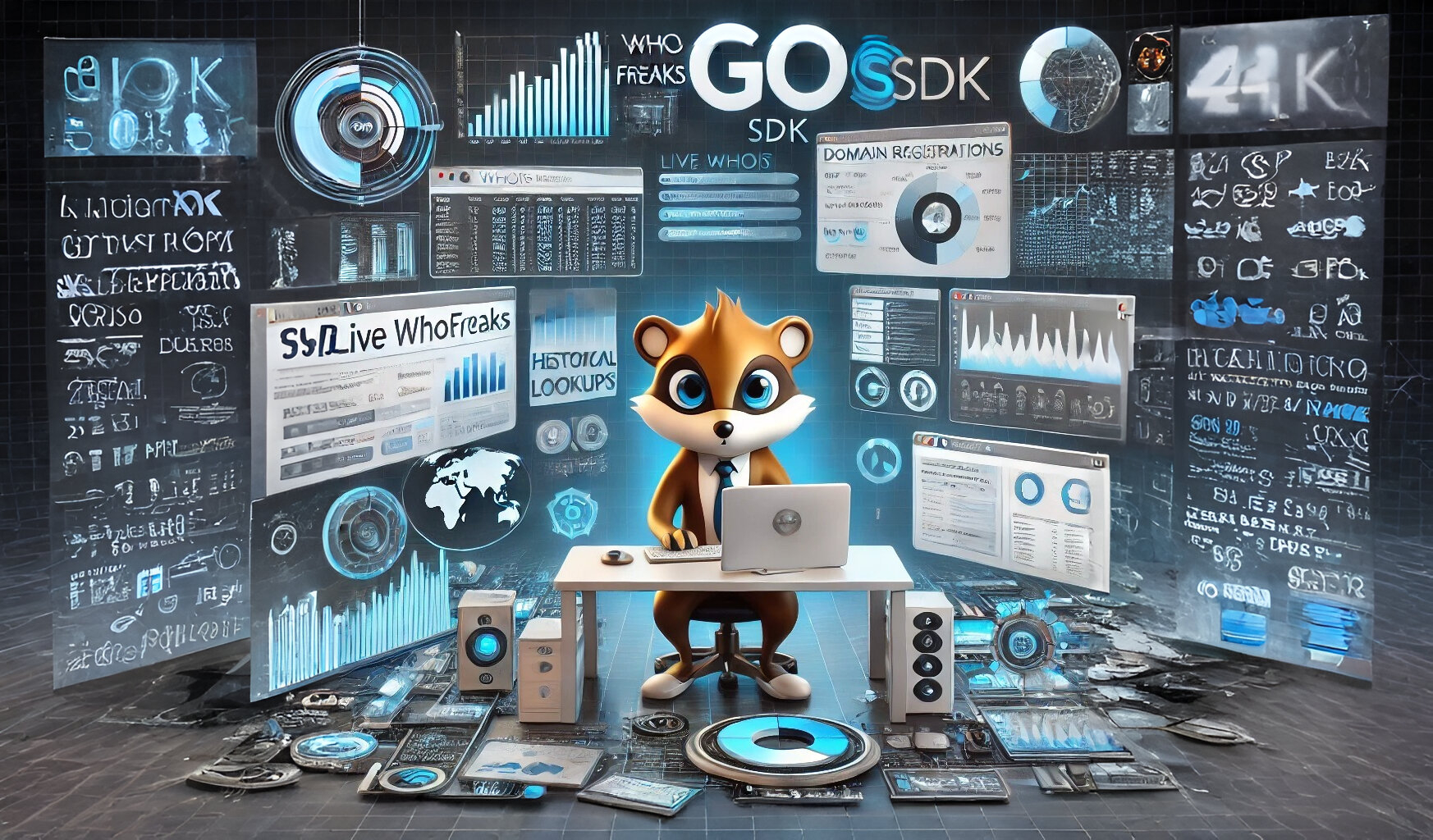
Go Domain Availability Lookup SDK
Leverage this Go Lang-based SDK tool to easily check domain availability through the WhoisFreaks API and integrate domain registration checks.
pkg.go.dev