Developer Libraries
Third-party Integrations
Best WHOIS Lookup SDK: Integrate WhoisFreaks APIs using Go
The WHOIS Go Lang SDK of WhoisFreaks provides powerful WHOIS lookup capabilities, enabling developers to retrieve live and historical WHOIS data. With this feature, you can access detailed domain registration information, track ownership history, and gain insights into domain changes, making it a key tool for domain analysis and cybersecurity.
How to perform WHOIS lookup(s) in go?
Go Whois SDK package allows you to perform various types of WHOIS lookups, including live, historical, and reverse lookups.
To utilize the WhoisFreaks Go SDK for WHOIS lookups, follow these steps:
-
Whois Authentication Setup
To authenticate your API requests, set your API key using the SetAPIKey method provided by the SDK. This method sets the global API key to the specified value.
bash Copyfunc SetAPIKey(key string)
Parameter:
- key: A string representing the API key to be set.
Example Usage:
go Copypackage main import ( "github.com/WhoisFreaks/whoisfreaks/whois" ) func main() { whois.SetAPIKey("your_api_key") // Your code here }
Replace "your_api_key" with your actual API key.
-
Whois Live Lookup
GetLiveResponse retrieves live WHOIS information for a specific domain using the WhoisFreaks API.
go Copyfunc GetLiveResponse(domain string) (*modal.DomainInfo, *modal.Error)
Parameter:
- domain: The domain name for which live WHOIS information is requested (e.g., "example.com").
Returns:
- *modal.DomainInfo: A pointer to a DomainInfo struct containing live domain information.
- *modal.Error: A pointer to an Error struct if there is an API error, or nil if the request is successful.
Example Usage:
go Copypackage main import ( "fmt" "log" "github.com/WhoisFreaks/whoisfreaks/whois" ) func main() { whois.SetAPIKey("your_api_key") domain := "example.com" result, err := whois.LiveLookup(domain) if err != nil { log.Fatal(err) } fmt.Println(result) }
Replace "example.com" with the domain you wish to query.
-
Whois Bulk Live Lookup
GetBulkLiveResponse retrieves live WHOIS information for multiple domains in bulk using the WhoisFreaks API.
go Copyfunc GetBulkLiveResponse(domains []string) (*modal.BulkDomainInfo, *modal.Error)
Parameter:
- domains: A slice of strings containing the domain names for which live WHOIS information is requested.
Returns:
- *modal.BulkDomainInfo: A pointer to a BulkDomainInfo struct containing live domain information for the bulk request.
- *modal.Error: A pointer to an Error struct if there is an API error, or nil if the request is successful.
Example Usage:
go Copypackage main import ( "fmt" "log" "github.com/WhoisFreaks/whoisfreaks/whois" ) func main() { whois.SetAPIKey("your_api_key") domains := []string{"example1.com", "example2.com", "example3.com"} results, err := whois.BulkLiveLookup(domains) if err != nil { log.Fatal(err) } for _, result := range results { fmt.Println(result) } }
Replace the domain names in the domains slice with the domains you wish to query.
-
Whois Historical Lookup
GetHistoricalResponse retrieves historical WHOIS information using the WhoisFreaks API.
go Copyfunc GetHistoricalResponse(domain string) (*modal.HistoricalDomainInfo, *modal.Error)
Parameter:
- domain: The domain name for which historical WHOIS information is requested (e.g., "example.com").
Returns:
- *modal.HistoricalDomainInfo: A pointer to a HistoricalDomainInfo struct containing historical domain information.
- *modal.Error: A pointer to an Error struct if there is an API error, or nil if the request is successful.
Example Usage:
go Copypackage main import ( "fmt" "log" "github.com/WhoisFreaks/whoisfreaks/whois" ) func main() { whois.SetAPIKey("your_api_key") domain := "example.com" result, err := whois.HistoricalLookup(domain) if err != nil { log.Fatal(err) } fmt.Println(result) }
Replace "example.com" with the domain you wish to query.
-
Whois Reverse Lookup
GetReverseResponse performs a reverse whois lookup using the WhoisFreaks API.
go Copyfunc GetReverseResponse(keyword, email, company, owner, page string) (*modal.ReverseDomainInfo, *modal.Error)
Parameters:
- keyword: The keyword to search for in domain records.
- email: The email address to search for in domain records.
- company: The company name to search for in domain records.
- owner: The owner name to search for in domain records.
- page: The optional page number for paginated results. Leave empty for the first page.
Returns:
- *modal.ReverseDomainInfo: A pointer to a ReverseDomainInfo struct containing reverse whois information.
- *modal.Error: A pointer to an Error struct if there is an API error, or nil if the request is successful.
Example Usage:
go Copypackage main import ( "fmt" "log" "github.com/WhoisFreaks/whoisfreaks/whois" ) func main() { whois.SetAPIKey("your_api_key") keyword := "whoisfreaks" email := "email@example.com" owner := "elon" company := "google" page := "2" result, err := whois.GetReverseResponse(keyword, email, company, owner, page) if err != nil { log.Fatal(err) } fmt.Println(result) }
Replace keyword, email, owner, company, and page with the desired keyword, email address, owner name, company name, and page number, respectively.
-
Whois Reverse Mini Lookup
GetReverseMiniResponse performs a reverse whois lookup in mini mode using the WhoisFreaks API.
go Copyfunc GetReverseMiniResponse(keyword, email, company, owner, page string) (*modal.ReverseMiniDomainInfo, *modal.Error)
Parameters:
- keyword: The keyword to search for in domain records.
- email: The email address to search for in domain records.
- company: The company name to search for in domain records.
- owner: The owner name to search for in domain records.
- page: The optional page number for paginated results. Leave empty for the first page.
Returns:
- *modal.ReverseMiniDomainInfo: A pointer to a ReverseMiniDomainInfo struct containing reverse whois information in mini mode.
- *modal.Error: A pointer to an Error struct if there is an API error, or nil if the request is successful.
Example Usage:
go Copypackage main import ( "fmt" "log" "github.com/WhoisFreaks/whoisfreaks/whois" ) func main() { whois.SetAPIKey("your_api_key") keyword := "whoisfreaks" email := "email@example.com" owner := "elon" company := "google" page := "2" result, err := whois.GetReverseMiniResponse(keyword, email, company, owner, page) if err != nil { log.Fatal(err) } fmt.Println(result) }
Replace keyword, email, owner, company, and page with the desired keyword, email address, owner name, company name, and page number, respectively.
Click here for instructions on performing DNS lookups with the Go SDK.
Click here to find out how to check domain availability using the Go SDK.
Click here if you want to learn how to perform SSL certificate lookups using the Go SDK.
Access the official WhoisFreaks Go SDK documentation for detailed WHOIS lookup functionality.
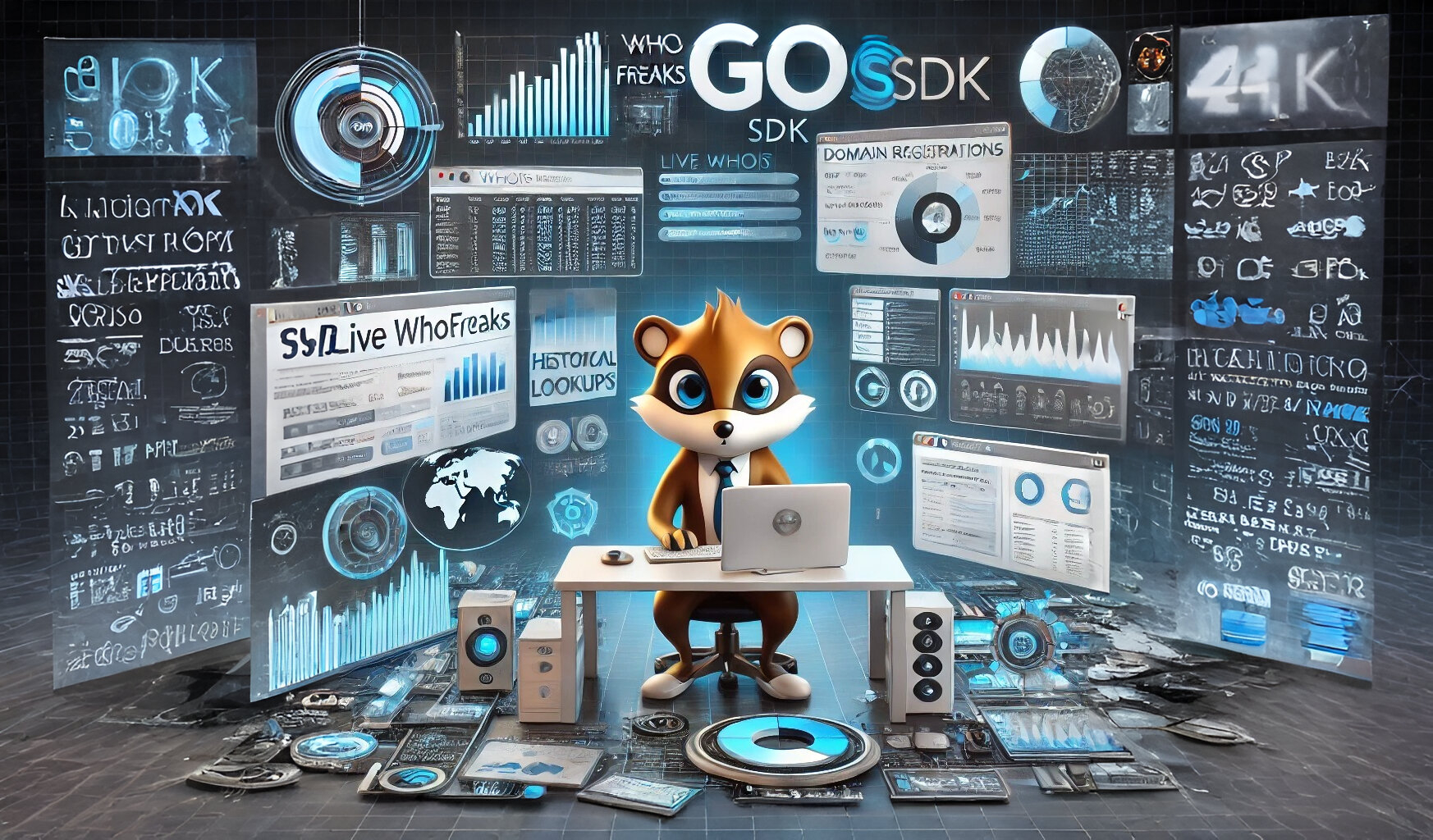
Go WHOIS Lookup SDK
Leverage this Go Lang-based SDK tool to interact with the WhoisFreaks WHOIS API seamlessly for live and historical domain data retrieval.
pkg.go.dev